High-Level Design vs Low-Level Design
When building a software system, architects and developers break down the design into two distinct levels: High-Level Design (HLD) and Low-Level Design (LLD). These two stages of design provide different perspectives and details of the system, helping developers visualize the overall architecture and also implement finer details at the component level. Understanding the difference between HLD and LLD is crucial for building scalable, maintainable, and efficient systems.
In this tutorial, we will dive into the concepts of HLD and LLD, explore their differences, and explain how they complement each other in designing modern software systems.
What is High-Level Design (HLD)?
High-Level Design (HLD) provides a bird’s-eye view of the system’s architecture. It outlines the system’s structure, major components, and how they interact with each other. Think of HLD as the blueprint for the overall system, which gives stakeholders a clear picture of how the system will function without going into the nitty-gritty details.
Key Aspects of HLD
- System Architecture: HLD focuses on defining the architecture of the system. This includes identifying the major components (like databases, servers, external services), how they will interact, and the technology stack used (e.g., web servers, databases, and communication protocols).
- Data Flow and Integration: HLD explains how data will flow between different components. It identifies how data is processed, stored, and communicated across different modules, and outlines any external integrations like third-party services (payment gateways, APIs, etc.).
- Technology Choices: HLD defines the high-level technology choices such as programming languages, database management systems, web frameworks, and cloud platforms. For instance, HLD might specify that the system will use a PostgreSQL database, Nginx as a web server, and an AWS cloud infrastructure.
- Non-functional Requirements: HLD also addresses non-functional aspects such as system scalability, availability, security, and performance. This helps guide the choices made in the lower levels of design.
Example of HLD
Imagine you are building an e-commerce platform. The HLD would include details like:
- Web Servers: Nginx or Apache HTTP Server to handle incoming requests.
- Database: MySQL or PostgreSQL for managing customer and product data.
- Cache: Redis or Memcached to cache frequently accessed product information.
- API Gateway: Handles communication between the front end and backend services.
- Third-party Integrations: Payment gateways (Stripe, PayPal) for handling transactions, and logistics APIs for managing shipping.
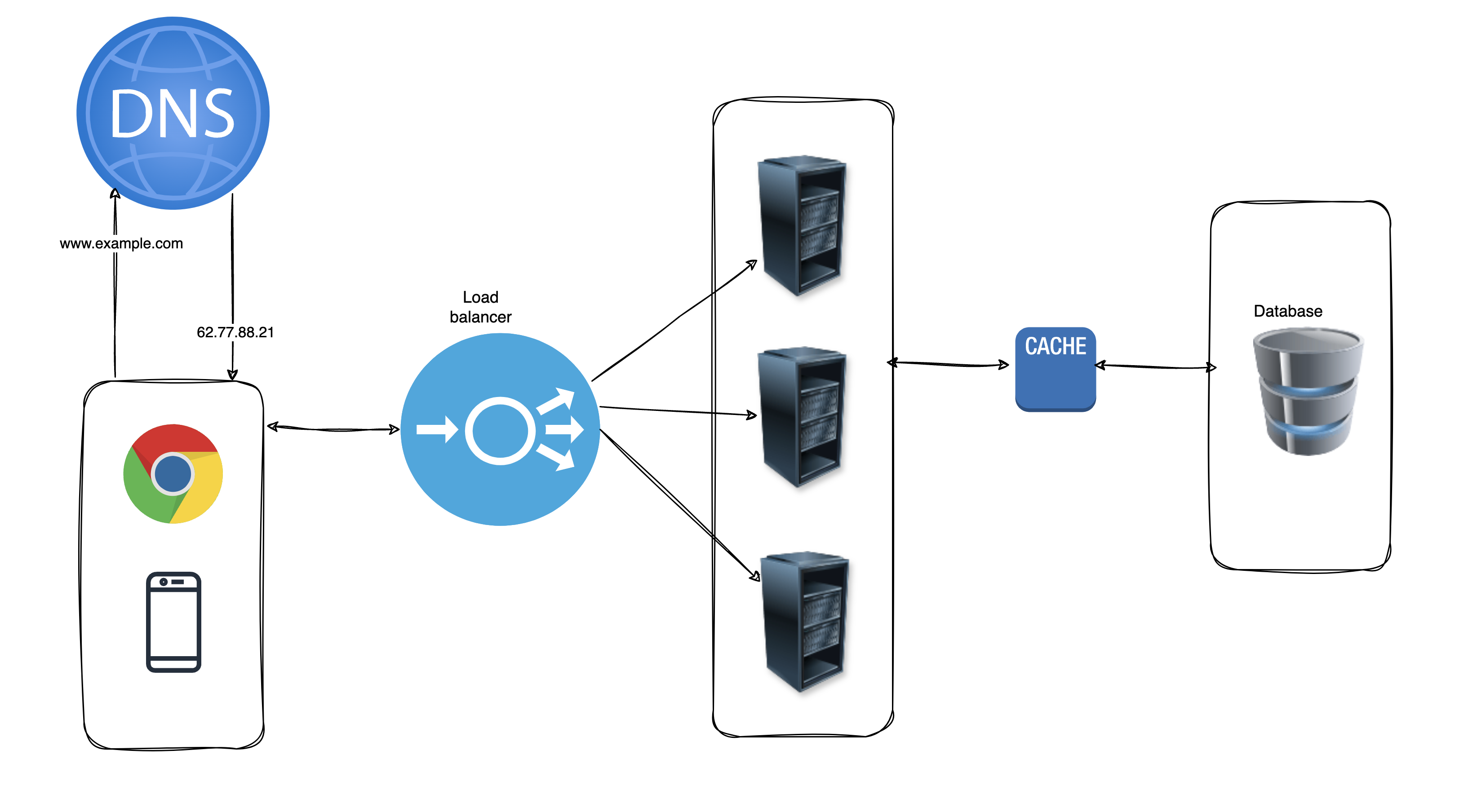
The HLD provides a complete picture of the system’s major components and how they work together, without getting into specific details like database schema or API endpoints.
What is Low-Level Design (LLD)?
Low-Level Design (LLD) zooms in on the details of the system. It breaks down each component defined in the HLD into smaller subcomponents and describes how they will be implemented. LLD focuses on the internal workings of each module, providing developers with the necessary information to write the actual code.
Key Aspects of LLD
- Detailed Component Design: LLD explains the design of each component in detail. This includes class diagrams, database schemas, and detailed flowcharts. LLD describes how each function and module will work, including algorithms, data structures, and control flow.
- Database Design: While HLD might specify the use of a relational database like MySQL, LLD will provide the actual database schema, including the tables, relationships, indexes, and queries. For example, it might describe a table for
Users
with columns foruser_id
,name
,email
, andpassword
. - API Design: If the system includes APIs, LLD will detail the API endpoints, request and response formats, and how different components communicate with each other. It defines the exact input and output for each API, along with error handling mechanisms.
- Algorithms and Data Structures: LLD describes the specific algorithms and data structures that will be used to implement functionality. For example, it might outline that a binary search algorithm will be used for searching through a list of products.
- Class and Sequence Diagrams: In object-oriented systems, LLD includes class diagrams that define the attributes and methods of each class. Sequence diagrams show how different objects interact with each other at runtime.
Example of LLD
Continuing with the e-commerce example, the LLD would include:
- Database Schema: A detailed schema of the
Products
table, including columns likeproduct_id
,name
,price
,category
, andstock_quantity
. It might also include relationships between tables such asOrders
,Customers
, andPayments
. - API Endpoint: For example, an API for retrieving product information:
- Endpoint:
GET /api/products/{id}
- Request:
{ "id": 123 }
- Response:
{ "product_id": 123, "name": "Laptop", "price": 999.99 }
- Endpoint:
- Class Design: For a
Product
class, you might define attributes likeproduct_id
,name
,price
, and methods likeupdatePrice()
,checkStock()
, etc. - Sequence Diagram: A sequence diagram showing how a user interacts with the product catalog. For instance, the user sends a request to the product API, which queries the database and returns the product information.
LLD ensures that every component has a clear plan for implementation and that developers know exactly what needs to be built.
For a generic example of a simple system, I will describe a class diagram for a basic Library Management System with classes like Book
, LibraryMember
, Loan
, and Librarian
.
Class Diagram Description
@startuml
class Book {
+ ISBN : String
+ title : String
+ author : String
+ publicationYear : int
+ checkout()
+ returnBook()
}
class LibraryMember {
+ memberId : String
+ name : String
+ phoneNumber : String
+ register()
+ borrowBook()
+ returnBook()
}
class Librarian {
+ employeeId : String
+ addBook()
+ removeBook()
}
LibraryMember <|-- Librarian
class Loan {
+ loanId : String
+ loanDate : Date
+ dueDate : Date
+ calculateDueDate()
+ checkOverdue()
}
LibraryMember "1" --o "0..*" Book : borrows
Loan --> Book
Loan --> LibraryMember
@enduml
Relationships
LibraryMember
andBook
: Aggregation (A member can borrow books)Librarian
andLibraryMember
: Inheritance (Librarian is a specialized type of LibraryMember)Loan
connectsBook
andLibraryMember
(composition, since loan cannot exist without a book or a member)
HLD vs LLD: Key Differences
Scope
- HLD: Overall system architecture and component interactions.
- LLD: Internal design and implementation of individual components.
Level of Detail
- HLD: High-level overview, no detailed implementation.
- LLD: Detailed design, including algorithms, classes, and data structures.
Audience
- HLD: System architects and stakeholders.
- LLD: Developers responsible for implementation.
Design Artifacts
- HLD: System architecture diagrams, data flow diagrams, tech stack choices.
- LLD: Class diagrams, sequence diagrams, database schemas, pseudocode.
Timing in Lifecycle
- HLD: Created early to define architecture.
- LLD: Created after HLD, focusing on implementation details.
How HLD and LLD Complement Each Other
While HLD provides a high-level overview, LLD fills in the details necessary for actual implementation. Together, they ensure that the system design is both conceptually sound and practically implementable.
- HLD lays the foundation by defining the major components, the technology stack, and how the system will handle non-functional requirements like scalability and availability.
- LLD builds on this foundation by specifying how each component will work, how data will be structured, and how individual functions will be implemented.
For example, HLD might specify that the system needs to handle 100,000 concurrent users, while LLD will outline how the load balancing mechanism will be implemented to ensure smooth scaling under this load.
Conclusion
High-Level Design (HLD) and Low-Level Design (LLD) are two essential stages of the system design process. HLD provides a high-level view of the system’s architecture, while LLD dives into the details of each component’s implementation. Together, they help ensure that systems are designed to be scalable, maintainable, and efficient.
Understanding the difference between HLD and LLD, and how they complement each other, is crucial for software developers and system architects alike. By mastering both, you’ll be better equipped to design robust , scalable systems that meet both functional and non-functional requirements.
In your next project, make sure to give both HLD and LLD the attention they deserve, and you’ll find that the design process becomes much smoother and the system much easier to implement and maintain.