How to use PIP - Pythons Package Manager?
pip is Python’s standard package manager, allowing you to easily install, upgrade, and remove libraries and tools from the Python ecosystem. With a simple command, pip enables you to access thousands of Python packages from the Python Package Index (PyPI). Whether you need libraries for web development, machine learning, or data analysis, pip streamlines the process, making package management fast and easy.
If you dont have PIP, Click here check "How to install PIP?"
What is covered in this document?
- Introduction to pip
- Checking pip Installation
- Basic Usage of pip
- Advanced pip Commands
- Managing Virtual Environments with pip
- Common Issues and Troubleshooting
- Best Practices for Using pip
- Conclusion
1. Checking pip Installation
Before diving into using pip, it's important to ensure that it is properly installed on your system. Open your terminal and type the following command:
pip --version
This will return the pip version installed on your machine, confirming that it's ready to use. If you see an error like command not found
, you’ll need to install pip first. Refer to the guide on installing pip for detailed instructions.
2. Basic Usage of pip
Installing Packages
The most common use of pip is installing Python packages. To install a package, run the following command:
pip install package_name
For example, to install the popular requests
library, you would run:
pip install requests
This downloads and installs the package along with its dependencies.
Upgrading Packages
To upgrade an already installed package, you can use the --upgrade
flag:
pip install --upgrade package_name
For instance, if you want to upgrade the requests
package to the latest version:
pip install --upgrade requests
Uninstalling Packages
If you no longer need a package, you can easily remove it:
pip uninstall package_name
For example, to uninstall requests
:
pip uninstall requests
3. Advanced pip Commands
Listing Installed Packages
To see a list of all installed packages, use the pip list
command:
pip list
This will output a list of installed packages and their respective versions.
Freezing Package Versions
If you want to create a file that lists the exact versions of all installed packages (useful for sharing environments), you can use:
pip freeze > requirements.txt
This will save the current environment’s packages to a requirements.txt
file, which can be used to recreate the environment later.
Installing from a Requirements File
To install all packages listed in a requirements.txt
file, use:
pip install -r requirements.txt
This is commonly used in projects to ensure all dependencies are installed with the correct versions.
Installing Specific Package Versions
If you need to install a particular version of a package, specify the version like this:
pip install package_name==x.x.x
For example, to install version 2.25.1 of requests
:
pip install requests==2.25.1
4. Managing Virtual Environments with pip
What are Virtual Environments?
Virtual environments allow you to isolate Python projects so that different projects can use different package versions. This avoids version conflicts and ensures that your system remains clean.
Creating and Activating Virtual Environments
To create a virtual environment, use the following command:
python -m venv env_name
Replace env_name
with your desired environment name. To activate the virtual environment:
On macOS/Linux:
source env_name/bin/activate
Once activated, your terminal will show the environment name, and any pip commands will only affect that environment.
Using pip Within a Virtual Environment
Once inside a virtual environment, you can use pip as normal. Installing packages inside a virtual environment ensures they don’t interfere with system-wide packages.
5. Common Issues and Troubleshooting
Error 1: Could not find a version that satisfies the requirement
Explanation: This error occurs when the package or version you're trying to install isn’t compatible with your Python version or isn't available.
Solution: Double-check the Python version and the package's compatibility. You can also try searching for the correct version on PyPI.
Error 2: SSL Certificate Error
**Explanation: Sometimes pip encounters SSL errors while trying to install packages.
Solution: You can bypass SSL verification by adding the --trusted-host
option:
pip install --trusted-host pypi.org --trusted-host files.pythonhosted.org package_name
Error 3: Permissions Error
Explanation: This error typically occurs when trying to install packages globally without proper permissions.
Solution: Use sudo
to grant elevated permissions or install packages for the current user:
pip install --user package_name
6. Best Practices for Using pip
- Keep pip updated: Regularly update pip to benefit from the latest features and security fixes.
pip install --upgrade pip
- Use virtual environments: Always use virtual environments for isolated package management, especially when working on multiple projects.
- Lock dependencies: For larger projects, save the state of your environment using
pip freeze
and ensure consistent package versions withrequirements.txt
. - Clean up packages: Regularly check and uninstall unused packages to keep your environment clean and organized.
7. Conclusion
pip is a powerful tool that simplifies the process of managing Python packages. By learning the basic commands and best practices, you can efficiently install, upgrade, and remove libraries, manage virtual environments, and troubleshoot common errors. With pip, you’ll have the flexibility to work with any Python project, whether you're developing for the web, data science, or beyond.
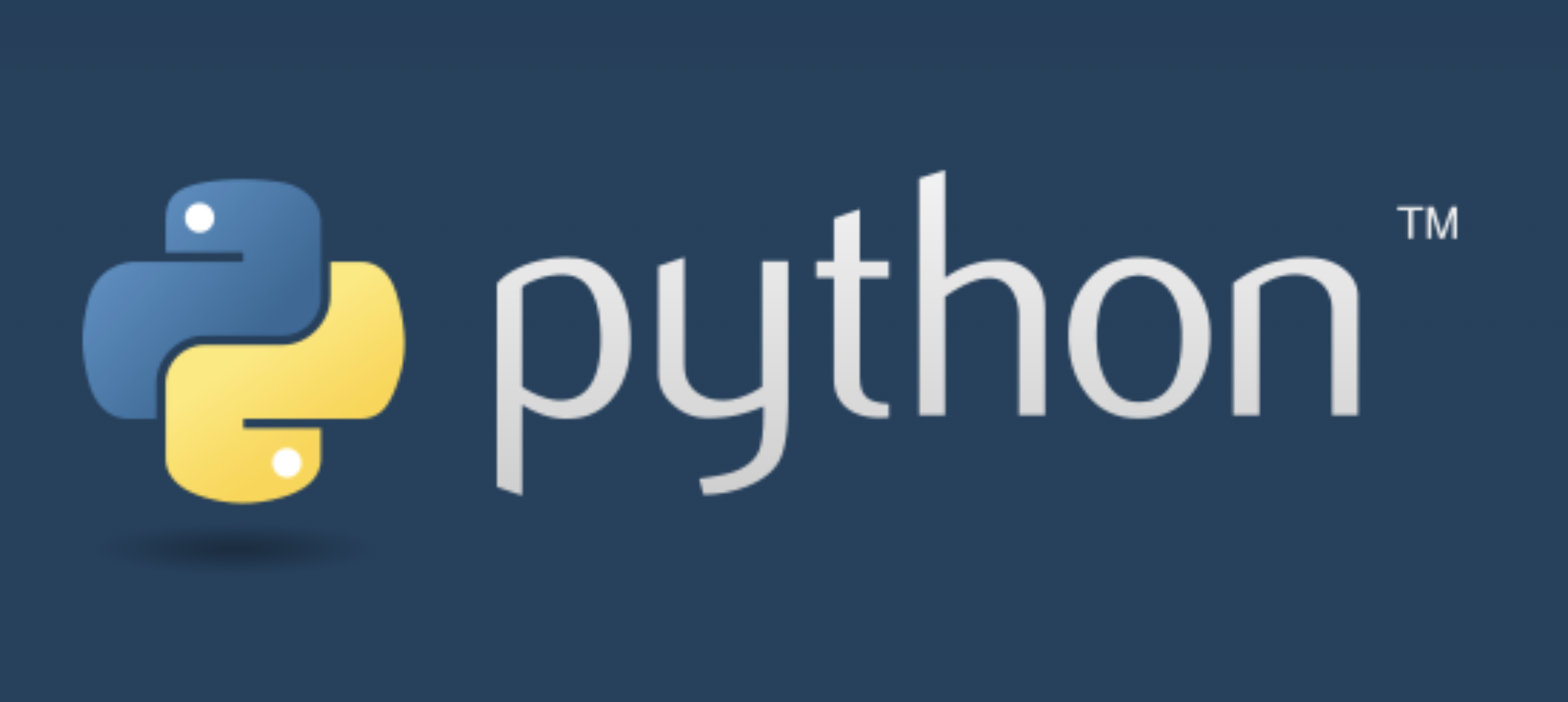